Exporting
A Scene
object can be exported to several file formats for use in rendereres, physics simulators etc.
The export(<filename>)
method will chose the appropriate exporter based on the filename extension:
from scene_synthesizer import procedural_scenes as ps
kitchen = ps.kitchen_island()
kitchen.export('/tmp/kitchen/kitchen.<obj,glb,stl,usd,urdf,..>')
Alternatively, the method can also return the raw data in the desired format (instead of writing the data to disk).
To do so, only pass file_type='<obj,usd,urdf,...>'
as the desired export format.
- Scene.export(fname=None, file_type=None, **kwargs)
Export scene as either: glb, gltf, obj, stl, ply, dict, json, urdf, usd, or usda.
- Parameters:
fname (str, optional) – Filename. If None, data is returned instead of written to disk. Defaults to None.
file_type (str, optional) – File format. If file_type is None, the type is deducted from the filename. If fname is None, file_type needs to be specified.
**kwargs (optional) – The export is highly configurable, each format has different parameters and flags. For details, see the format-specific docstrings in exchange.export_*.
- Returns:
If fname is None, data will be returned in the specified file_type.
- Return type:
str or bytes or dict (optional)
Note, that there are a lot of format-specific arguments that affect the exported result.
The easiest way to see them is to look up the docstring of the format-specific export function in the export
module.
In the following we show most of them.
Polygon Mesh
The scene object can be exported to the following common mesh representations: GLTF
, GLB
, OBJ
, STL
, PLY
, etc.
Note that this does not preserve articulations.
- scene_synthesizer.exchange.export.export_obj(scene, concatenate=True, **kwargs)
Export a scene as a Wavefront OBJ file.
This uses trimesh.exchange.obj.export_obj
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
concatenate (bool, optional) – Whether to concatenate all meshes into a single one. Note, that currently trimesh can’t export textured scenes. Defaults to True.
**include_normals (bool) – Include vertex normals in export. If None will only be included if vertex normals are in cache.
**include_color (bool) – Include vertex color in export
**include_texture (bool) – Include vt texture in file text
**return_texture (bool) – If True, return a dict with texture files
**write_texture (bool) – If True and a writable resolver is passed write the referenced texture files with resolver
**resolver (None or trimesh.resolvers.Resolver) – Resolver which can write referenced text objects
**digits (int) – Number of digits to include for floating point
**mtl_name (None or str) – If passed, the file name of the MTL file.
**header (str or None) – Header string for top of file or None for no header.
- Returns:
OBJ format output dict: Contains texture files that need to be saved in the same directory as the exported mesh: {file name : bytes}
- Return type:
str
- scene_synthesizer.exchange.export.export_glb(scene, **kwargs)
Export a scene as a binary GLTF (GLB 2.0) file.
This uses trimesh.exchange.gltf.export_glb
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
**extras (JSON serializable) – Will be stored in the extras field.
**include_normals (bool) – Whether to include vertex normals in output file. Defaults to None.
**unitize_normals (bool) – Whether to unitize normals. Defaults to False.
**tree_postprocessor (func) – Custom function to (in-place) post-process the tree before exporting.
**buffer_postprocessor (func) – Custom function to (in-place) post-process the buffer before exporting.
- Returns:
Exported result in GLB 2.0
- Return type:
bytes
- scene_synthesizer.exchange.export.export_gltf(scene, **kwargs)
Export a scene object as a GLTF directory.
This puts each mesh into a separate file (i.e. a buffer) as opposed to one larger file.
This uses trimesh.exchange.gltf.export_gltf
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
**include_normals (bool) – Whether to include vertex normals. Defaults to None.
**merge_buffers (bool) – Whether to merge buffers into one blob. Defaults to False.
**resolver (trimesh.resolvers.Resolver) – If passed will use to write each file.
**tree_postprocesser (None or callable) – Run this on the header tree before exiting. Defaults to None.
**embed_buffers (bool) – Embed the buffer into JSON file as a base64 string in the URI. Defaults to False.
- Returns:
Format: {file name : file data}
- Return type:
dict
- scene_synthesizer.exchange.export.export_stl(scene, ascii=False)
Export a scene as a binary or ASCII STL file.
This uses trimesh.exchange.stl.export_stl
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
ascii (bool, optional) – Binary or ASCII encoding. Defaults to False.
- Returns:
Scene mesh represented in binary or ASCII STL form.
- Return type:
str or bytes
- scene_synthesizer.exchange.export.export_ply(scene, **kwargs)
Export a scene in the PLY format.
This uses trimesh.exchange.ply.export_ply
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
**encoding (str) – PLY encoding: ‘ascii’ or ‘binary_little_endian’. Defaults to ‘binary’.
**vertex_normal (bool or None) – None or include vertex normals. Defaults to None.
**include_attributes (bool) – Defaults to True.
- Returns:
Scene mesh represented in binary or ASCII PLY form.
- Return type:
str or bytes
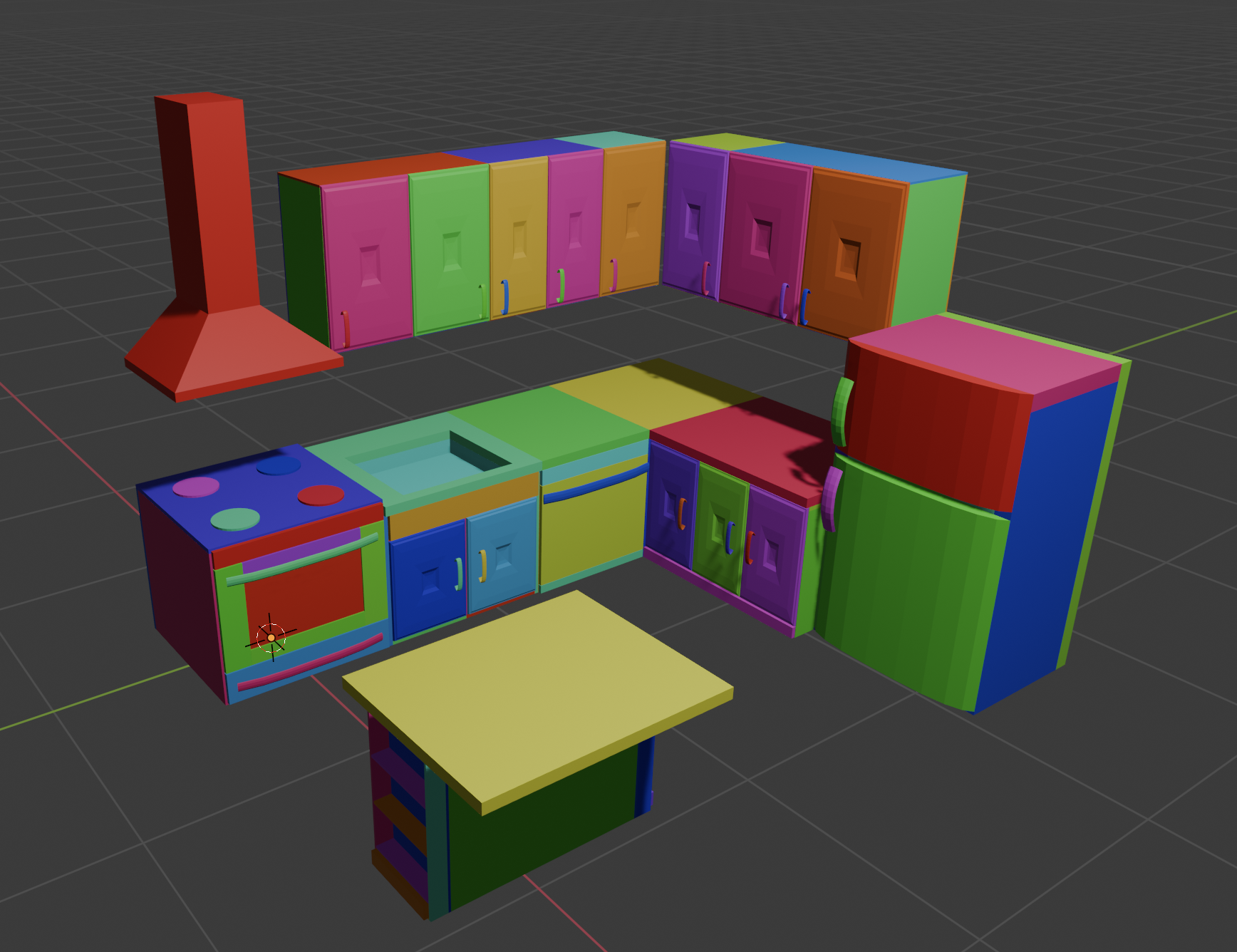
OBJ loaded in Blender.
JSON
Sometimes the scene data is required in a more parsable format. The JSON export serializes the scene into a dictionary containing the scene graph and geometry information.
- scene_synthesizer.exchange.export.export_json(scene, use_base64=False, include_metadata=True)
Export a scene to a JSON string.
Note, the resulting JSON file can be loaded again via scene_synthesizer.Scene.load().
- Parameters:
scene (scene_synthesizer.Scene) – Scene to be exported.
use_base64 (bool, optional) – Encode arrays with base64 or not. Defaults to False.
include_metadata (bool, optional) – Whether to include scene metadata. Defaults to True.
- Returns:
Scene as JSON string.
- Return type:
str
URDF
The URDF format is a popular XML specification in robotics.
- scene_synthesizer.exchange.export.export_urdf(scene, fname, folder=None, mesh_dir=None, use_absolute_mesh_paths=False, include_camera_node=False, include_light_nodes=False, separate_assets=False, write_mesh_files=False, write_mesh_file_type='obj', single_geometry_per_link=False, mesh_path_prefix=None, ignore_layers=None)
Export scene to one/multiple URDF files or return URDF data as string(s).
- Parameters:
scene (scene_synthesizer.Scene) – Scene description.
fname (str) – URDF filename or None. If None, the URDF data is returned as one or multiple strings.
folder (str, optional) – Only used if fname is None. The folder in which to export the URDF. Affects the location of textures. Defaults to None.
mesh_dir (str, optional) – Mesh directory to write polygon meshes to. Defaults to the directory part of fname or folder if fname is None.
use_absolute_mesh_paths (bool, optional) – If set to True will convert all mesh paths to absolute ones. Defaults to False.
mesh_path_prefix (str, optional) – Can be used to set file name prefix for meshes to “file://” or “package://”. Defaults to “”.
write_mesh_files (bool, optional) – If False, mesh file names in the URDF will point to the asset source files. If True, the meshes will be written to the URDF mesh directory. Defaults to True.
write_mesh_file_type (str, optional) – File type that will be used if write_mesh_files is True. Defaults to “obj”.
separate_assets (bool, optional) – If True, each asset in the scene will be exported to a separate URDF file, named according to its object identifier. Note: The scene itself will not be exported; each asset’s transformation will not be preserved. Defaults to False.
include_camera_node (bool, optional) – Whether to include a link for the camera node. Defaults to False.
include_light_nodes (bool, optional) – Whether to include a link for the light nodes. Defaults to False.
single_geometry_per_link (bool, optional) – If True will add only one visual/collision geometry per link. This creates a lot of links and fixed joints. Defaults to False.
ignore_layers (bool, optional) – If True will add all geometries as visual and collision geometries. If None, will it will be (num_layers < 2). Defaults to None.
- Raises:
ValueError – Raise an exception if mesh_dir is not absolute but use_absolute_mesh_paths=True.
- Returns:
If fname is None, will return the URDF data as a string. dict[str, str] (optional): If fname=None and separate_assets=True will return the additional assets as URDF strings in a dictionary.
- Return type:
str (optional)
To create a URDF file that is viewable in ROS RViz, use the following arguments:
from scene_synthesizer import procedural_scenes as ps
kitchen = ps.kitchen_island().colorize()
kitchen.export(
'/tmp/kitchen/kitchen.urdf',
use_absolute_mesh_paths=True,
mesh_path_prefix='file://')
And load it in RViz as follows. Make sure to set Global Options -> Fixed Frame
to the base frame of the scene, here world
.
roslaunch urdf_tutorial display.launch model:=/tmp/kitchen/kitchen.urdf
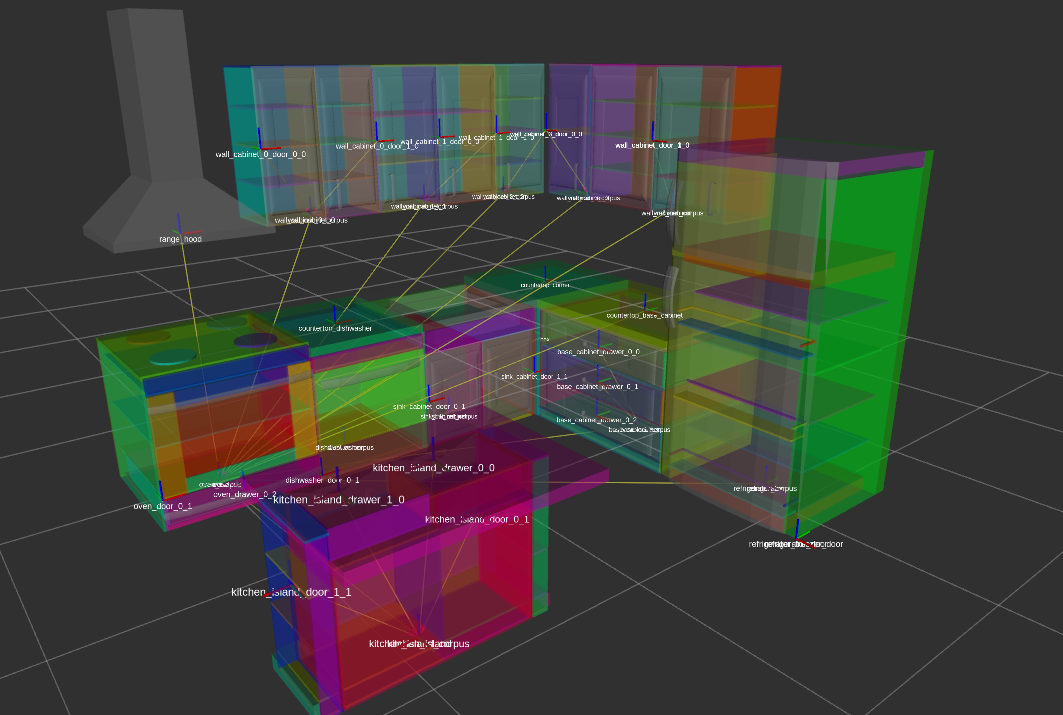
URDF loaded in RViz.
USD
The Universal Scene Description (USD) is a versatile file format widely used in rendering and simulation applications. For example, a USD file can be utilized in platforms like Isaac Sim or Isaac Lab. Additionally, the file can be inspected using the usdview tool.
- scene_synthesizer.exchange.export.export_usd(scene, fname, file_type='usd', folder=None, texture_dir='textures', export_materials=True, separate_assets=False, use_absolute_usd_paths=False, write_usd_object_files=True, include_light_nodes=False, ignore_layers=None, up_axis='Z', meters_per_unit=1.0, kilograms_per_unit=1.0, write_attribute_attached_state=False, write_semantic_labels_api=True)
Export scene to USD file or return USD data.
- Parameters:
scene (scene_synthesizer.Scene) – Scene description.
fname (str) – The USD filename or None. If None the USD stage is returned.
file_type (str, optional) – Either ‘usd’, ‘usda’, or ‘usdc’. Defaults to ‘usd’.
folder (str, optional) – Only used if fname is None. The folder in which to export the USD(s). Affects the location of textures. Defaults to None.
texture_dir (str, optional) – Directory where texture files will be written (relative to USD main file). Defaults to “textures”.
export_materials (bool, optional) – Whether to write out texture files. Defaults to True.
separate_assets (bool, optional) – If True, each asset in the scene will be exported to a separate USD file, named according to its object identifier. Defaults to False.
use_absolute_usd_paths (bool, optional) – If set to True will convert all referenced USD paths to absolute ones. Defaults to False.
write_usd_object_files (bool, optional) – Write USD files instead of referencing existing ones. Defaults to True.
include_light_nodes (bool, optional) – Whether to include a link for the light nodes. Defaults to False.
ignore_layers (bool, optional) – Whether to ignore scene layers. If None, scenes with one layer or less will ignore the layers. Defaults to None.
up_axis (str, optional) – Either ‘Y’ or ‘Z’. Defaults to “Z”.
meters_per_unit (float, optional) – Meters per unit. Defaults to 1.0.
kilograms_per_unit (float, optional) – Kilograms per unit. Defaults to 1.0.
write_attribute_attached_state (bool, optional) – Will write attribute ‘AttachedState’ for objects whose parent is not the world. Defaults to False.
write_semantic_labels_api (bool, optional) – Will write USDs SemanticsLabelsAPI based on scene’s metadata ‘semantic_labels’. Defaults to True.
- Returns:
If fname is None, will return the USD stage (created in memory). dict[str, Usd.Stage] (optional): If fname=None and separate_assets=True will return the additional assets as USD stages in a dictionary.
- Return type:
Usd.Stage (optional)
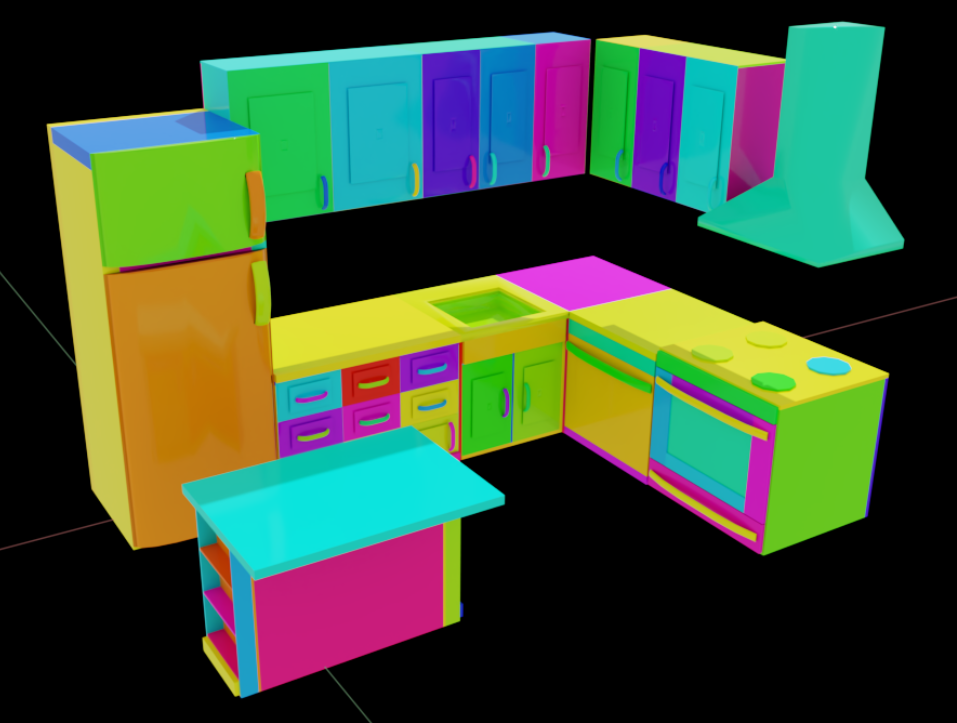
USD loaded in Isaac Sim.